python题目Write a function that computes and returns the sum o
来源:学生作业帮 编辑:搜狗做题网作业帮 分类:综合作业 时间:2024/05/18 05:10:18
python题目
Write a function that computes and returns the sum of the digits in an integer. Use the following function header:
def sum_digits(number):
For
example sum_digits(234) returns 9. Use the % operator to extract the
digits and the // operator to remove the extracted digit. For example,
to extract 4 from 234, use 234 % 10 (= 4).To remove 4 from 234, use
234//10 (= 23). Use a loop to repeatedly extract and remove the digits
until all the digits are extracted. Write a main function with the
header "def main():" that prompts the user to enter an
integer, calls the sum_digits function and then displays the results
returned by the function. The main function is called by writing
"main()". The template for the question is given below:
# Template starts here
def sum_digits(number):
# Write code to find the sum of digits in number and return the sum
def main():
# Write code to prompt the user to enter an integer, call sumDigits and display result
main()# Call to main function
# Template ends here
Sample Output
Enter a number: 123
Sum of digits: 6
Write a function that computes and returns the sum of the digits in an integer. Use the following function header:
def sum_digits(number):
For
example sum_digits(234) returns 9. Use the % operator to extract the
digits and the // operator to remove the extracted digit. For example,
to extract 4 from 234, use 234 % 10 (= 4).To remove 4 from 234, use
234//10 (= 23). Use a loop to repeatedly extract and remove the digits
until all the digits are extracted. Write a main function with the
header "def main():" that prompts the user to enter an
integer, calls the sum_digits function and then displays the results
returned by the function. The main function is called by writing
"main()". The template for the question is given below:
# Template starts here
def sum_digits(number):
# Write code to find the sum of digits in number and return the sum
def main():
# Write code to prompt the user to enter an integer, call sumDigits and display result
main()# Call to main function
# Template ends here
Sample Output
Enter a number: 123
Sum of digits: 6
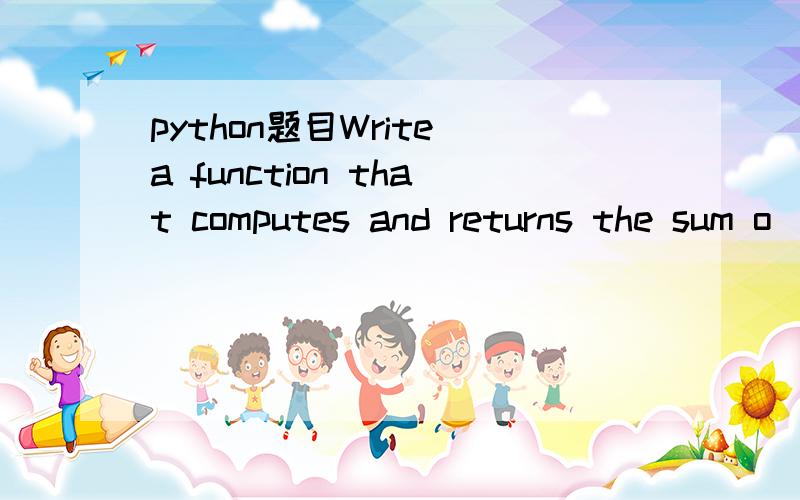
#!/usr/bin/env python
#-*- coding:utf-8 -*-
def sum_digits(number):
"""Return the sum of the number's digits"""
remain = number
sumn = 0
while remain>0:
sumn += remain % 10
remain = remain // 10
return sumn
def main():
"""To interact with user"""
while True :
numberstr = raw_input("Enter a number:")
if numberstr.isdigit():
result = sum_digits(int(numberstr))
print "Sum of digits: {0}".format(result)
break
else:
print "An int type you entered is not valid!"
if __name__ == '__main__':
main()
#-*- coding:utf-8 -*-
def sum_digits(number):
"""Return the sum of the number's digits"""
remain = number
sumn = 0
while remain>0:
sumn += remain % 10
remain = remain // 10
return sumn
def main():
"""To interact with user"""
while True :
numberstr = raw_input("Enter a number:")
if numberstr.isdigit():
result = sum_digits(int(numberstr))
print "Sum of digits: {0}".format(result)
break
else:
print "An int type you entered is not valid!"
if __name__ == '__main__':
main()
英语翻译The member function returns a reverse iterator that poin
英语翻译Write a Windows form application that returns the differ
新手python问题Write a function called digit_sumthat takes a posi
英语翻译Write a function that takes three arguments:a character
英语翻译Write a function that dynamically allocates an array of
求python大神,题目是write a program to keep track of conference att
一道高数题 Find A and B given that the function f(x) is different
英语翻译1.The home station function temporarily closes!2.Returns
数学题中write a simpler function agrees with the given function
Given that A is the sum ofthe square of 3 and the square of
Write a program which asks the user for four numbers(Python)
suppose that f is a continuously differentiable function and